Web - mesh of edges
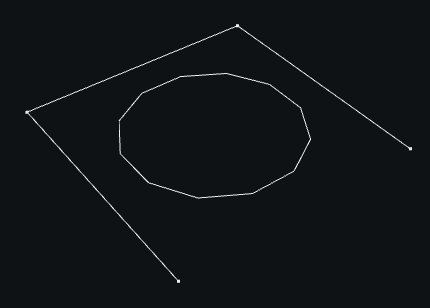
-
class
Web
(points=None, edges=None, tracks=None, groups=None, options=None)[source] set of bipoint edges, used to represent wires this definition is very close to the definition of Mesh, but with edges instead of triangles
Note
a Wire instance can contain non-connex geometries (like many separated outlines, called islands), or even non-linear meshing (like intersecting curves). In the purpose of part design, many functions may need more regular characteristics, so checking methods exists and it is up to the user to ensure the mesh do provide them when calling the demanding functions
-
points
typedlist of vec3 for points
-
edges
typedlist of couples for edges, the couple is oriented (meanings of this depends on the usage)
-
tracks
typedlist of integers giving the group each line belong to
-
groups
custom information for each group
-
options
custom informations for the entire web
-
own
(**kwargs) Self Return a copy of the current mesh, which attributes are referencing the original data or duplicates if demanded
Example
>>> b = a.own(points=True, faces=False) >>> b.points is a.points False >>> b.faces is a.faces True
-
option
(**kwargs) self Update the internal options with the given dictionary and the keywords arguments. This is only a shortcut to set options in a method style.
-
transform
(trans) Self Apply the transform to the points of the mesh, returning the new transformed mesh
-
mergeclose
(limit=None) dict Merge points below the specified distance, or below the precision return a dictionary of points remapping {src index: dst index}
O(n) implementation thanks to hashing
-
mergepoints
(merges) self [source] merge points with the merge dictionnary {src index: dst index} merged points are not removed from the buffer.
-
mergegroups
(defs=None, merges=None) self Merge the groups according to the merge dictionary The new groups associated can be specified with defs The former unused groups are not removed from the buffer and the new ones are appended
If merges is not provided, all groups are merged, and defs is the data associated to the only group after the merge
-
strippoints
() list [source] remove points that are used by no faces, return the reindex list. if used is provided, these points will be removed without usage verification
return a table of the reindex made
-
stripgroups
() list Remove groups that are used by no faces. return the reindex list.
-
finish
() self - Finish and clean the mesh
- note that this operation can cost as much as other transformation operation job done
- mergeclose
- strippoints
- stripgroups
- check
-
isvalid
() Return true if the internal data is consistent (all indices referes to actual points and groups)
-
pointnear
(point: dvec3) int Return the nearest point the the given location
-
pointat
(point: dvec3, neigh=1e-13) int Return the index of the first point at the given location, or None
-
group
(quals) Self [source] extract a part of the mesh corresponding to the designated groups.
- Groups can be be given in either the following ways:
a set of group indices
This can be useful to combine with other functions. However it can be difficult for a user script to keep track of which index correspond to which created group
an iterable of group qualifiers
This is the best way to designate groups, and is meant to be used in combination with
self.qual()
. This mode selects every group having all the input qualifiers
Example
>>> # create a mesh with only the given groups >>> mesh.group({1, 3, 8, 9}) <Mesh ...>
>>> # create a mesh with all the groups having the following qualifiers >>> mesh.group(['extrusion', 'arc']) <Mesh ...>
-
replace
(mesh, groups=None) self [source] replace the given groups by the given mesh. If groups is not specified, it will take the matching groups (with same index) in the current mesh
-
qualify
(*quals, select=None, replace=False) self Set a new qualifier for the given groups
Parameters: - quals – the qualifiers to enable for the selected mesh groups
- select (iterable) – if specified, only the groups having all those qualifiers will be added the new qualifiers
- replace (bool) – if True, the qualifiers in select will be removed
Example
>>> pool = meshb.qualify('part-a') + meshb.qualify('part-b') >>> set(meshb.faces) == set(pool.group('part-b').faces) True
>>> chamfer(mesh, ...).qualify('my-group', select='chamfer', replace=True) >>> mesh.group('my-group') <Mesh ...>
-
qualified_indices
(quals) Yield the faces indices when their associated group are matching the requirements
-
qualified_groups
(quals) Yield the groups indices when they are matching the requirements
-
maxnum
() float Maximum numeric value of the mesh, use this to get an hint on its size or to evaluate the numeric precision
-
precision
(propag=3) float Numeric coordinate precision of operations on this mesh, allowed by the floating point precision
-
surface
() float [source] return the surface enclosed by the web if planar and is composed of loops (else it has no meaning)
-
barycenter_points
() dvec3 Barycenter of points used
-
usepointat
(point, neigh=1e-13) int Return the index of the first point in the mesh at the location. If none is found, insert it and return the index
-
edgepoints
(e) tuple [source] tuple of the points for edge
e
the edge can be given using its index in
self.edges
or using a tuple of point idinces
-
edgedirection
(e) dvec3 [source] direction of edge e
the edge can be given using its index in
self.edges
or using a tuple of point idinces
-
extremities
() set [source] return the points that are used once only (so at wire terminations) 1D equivalent of Mesh.outlines()
-
groupextremities
() Wire [source] return the extremities of each group. 1D equivalent of Mesh.groupoutlines()
On a frontier between multiple groups, there is as many points as groups, each associated to a group.
-
frontiers
(*args) Wire [source] return a Wire of points that split the given groups appart.
The arguments are groups indices or lists of group qualifiers (as set in
qualify()
). If there is one only argument it is considered as as list of arguments.- if no argument is given, then return the frontiers between every groups
- to include the groups extremities that are on the group border but not at the frontier with an other group, add
None
to the group set
Example
>>> w = Web([...], [uvec2(0,1), uvec2(1,2)], [0, 1], [...]) >>> w.frontiers(0,1).indices [1]
>>> # equivalent to >>> w.frontiers({0,1}).indices [1]
>>> w.frontiers(0,None).indices [0]
-