Wire - mesh of points, or suite of points
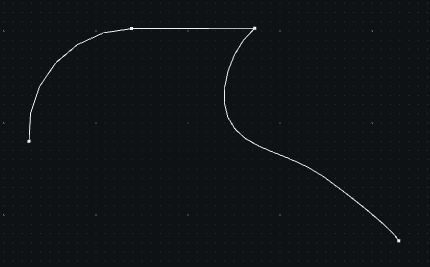
-
class
Wire
(points=None, indices=None, tracks=None, groups=None, options=None)[source] This class defines a mesh of points, used for two purposes:
- curves, wires, paths, storing the ordered suite of point indices
- cloud points, points extracted from other mesh, storing the unordered points indices
Most of the methods of
Wire
are intended for the first use case.conventions:
- A curve is considered closed (or to be a loop) when its final index is the same as the first.
- tracks are matching indices, giving a group each index. But for curves,
track[i]
gives a group for edge(indices[i], indices[i+1])
-
points
points buffer
-
indices
indices of the line’s points in the buffer
-
tracks
group index for each point in indices it can be used to associate groups to points or to edges (if to edges, then take care to still have as many track as indices)
-
groups
data associated to each point (or edge)
-
options
custom informations for the entire wire
-
own
(**kwargs) Self Return a copy of the current mesh, which attributes are referencing the original data or duplicates if demanded
Example
>>> b = a.own(points=True, faces=False) >>> b.points is a.points False >>> b.faces is a.faces True
-
option
(**kwargs) self Update the internal options with the given dictionary and the keywords arguments. This is only a shortcut to set options in a method style.
-
transform
(trans) Self Apply the transform to the points of the mesh, returning the new transformed mesh
-
mergeclose
(limit=None)[source] merge close points ONLY WHEN they are already linked by an edge. the meaning of this method is different than
Web.mergeclose()
-
mergepoints
(merges) self [source] merge points with the merge dictionnary {src index: dst index} merged points are not removed from the buffer.
-
mergegroups
(defs=None, merges=None) self Merge the groups according to the merge dictionary The new groups associated can be specified with defs The former unused groups are not removed from the buffer and the new ones are appended
If merges is not provided, all groups are merged, and defs is the data associated to the only group after the merge
-
strippoints
()[source] remove points that are used by no edge if used is provided, these points will be removed without usage verification
no reindex table is returned as its generation costs more than the stripping operation
-
stripgroups
() list Remove groups that are used by no faces. return the reindex list.
-
finish
() self - Finish and clean the mesh
- note that this operation can cost as much as other transformation operation job done
- mergeclose
- strippoints
- stripgroups
- check
-
pointnear
(point: dvec3) int Return the nearest point the the given location
-
pointat
(point: dvec3, neigh=1e-13) int Return the index of the first point at the given location, or None
-
group
(groups)[source] extract a part of the mesh corresponding to the designated groups.
- Groups can be be given in either the following ways:
a set of group indices
This can be useful to combine with other functions. However it can be difficult for a user script to keep track of which index correspond to which created group
an iterable of group qualifiers
This is the best way to designate groups, and is meant to be used in combination with
self.qual()
. This mode selects every group having all the input qualifiers
Example
>>> # create a mesh with only the given groups >>> mesh.group({1, 3, 8, 9}) <Mesh ...>
>>> # create a mesh with all the groups having the following qualifiers >>> mesh.group(['extrusion', 'arc']) <Mesh ...>
-
surface
() float [source] return the surface enclosed by the web if planar and is a loop (else it has no meaning)
-
barycenter_points
() dvec3 Barycenter of points used
-
normal
() dvec3 [source] return an approximated normal to the curve as if it was the outline of a flat surface. if this is not a loop the result is undefined.
-
vertexnormals
(loop=False)[source] return the opposed direction to the curvature in each point this is called normal because it would be the normal to a surface whose section would be that wire